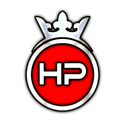
Remove Unwanted Spaces From String using Java
Remove Unwanted Spaces From String
Hi friends. Welcome to HP Kingdom.
This is a article is how to remove Unwanted spaces from a string Given a string using java, remove all spaces from it.
For example “ I like hpkingdom.com” should be converted to “I like hpkingdom.com” should be converted.
To right and ignore or remove the spaces while traversing. We need to keep track of two indexes, one for current character being read and other for current index in output.
Given 3 Methods:
- Remove Unwanted Space Using String (Basic Method).
- Remove extra white spaces between words using regular expression.
- Replace multiple spaces with single space using StringBuiffer.
Remove extra spaces given a String Example: Input : I like hpkingdom.com output:I like hpkingdom.com
1.Remove Unwanted Spaces Using String (Basic Method).
- using String
- Char Array
package hpkingdom.com; public class RemoveUnwantedSpace { public static void main(String[] args) { String s = " I Like hpkingdom.com "; char c[] = s.toCharArray(); String ss = ""; for (int i=0; i<c.length; i++) { if (c[i]==' ') { } else { ss=ss+c[i]; if (c[i+1]==' '){ ss=ss+" "; } } } System.out.println("Removed Spaces: " +ss); } }Output:
Removed Spaces: I Like hpkingdom.com
2.Remove extra white spaces between words with regular expression
Using regular expression, to replace 2 or more white spaces with single space, is also a good solution. We are using regex pattern as “\\s+”.\s
matches a space, tab, new line, carriage return, form feed or vertical tab.+
says one or more occurrences.
package hpkingdom.com; public class RemoveUnwantedSpace { public static void main(String[] args) { String str = "I Like hpkingdom.com "; String removespaces = str.replaceAll("\\s+", " "); System.out.println( "Removed Spaces: " +removespaces ); } }
Output:
Removed Spaces: I Like hpkingdom.com
Note that this method will not trim the String. That means there might be single space at start at end of string, if original string has such white spaces at beginning or end.3.Replace multiple spaces with single space using StringBuiffer
StringBuiffer
can also help you to get string from uneven spaced strings. This is complete programmatic approach and may not be suitable for large strings.
package hpkingdom.com; import java.util.StringTokenizer; public class RemoveUnwantedSpace { public static void main(String[] args) { String name = " I Like hpkingdom.com "; StringTokenizer st = new StringTokenizer(name, " "); StringBuffer sb = new StringBuffer(); while(st.hasMoreElements()) { sb.append(st.nextElement()).append(" "); } String nameSpace = sb.toString(); //trim leading and trailing white spaces nameSpace = nameSpace .trim(); System.out.println( "Removed Spaces: "+nameSpace ); } }